Simply provides a simple, powerful and secure application programming interface (API) to work with the entities stored within. To use this document, you should have a basic familiarity with software development, web services, and Simply CRM.
The web service provides an easier way to integrate Simply with your other software systems.
The API call characteristics
REST – API is REST based, which means that all the communication between the client and the server can happen over HTTP in the form of GET or POST requests.
JSON – JSON is used to encode the response and the request.
Requests and responses – Your client application prepares and submits a service request to the API. The API processes the request and returns a response, and then the client application handles the response.
Committed automatically – Every operation that writes to a Simply object is committed automatically. This is analogous to the AUTOCOMMIT setting in SQL.
The API response
Response Object
All responses will have one of the following two formats.
If the request is processed successfully:
<code>Response{
success:Boolean=true
result:Object //The Operation Result object
}
</code>
If a failure occurs while processing the request:
<code>Reponse{
success:Boolean=false
error:ErrorObject
}
</code>
Error object
<code>ErrorObject{
errorCode:String //String representation of the error type
errorMessage:String //Error message from the api.
}
</code>
errorCode is a string representation of the error type.
Error handling
The response for any web service request is a JSON object. The object has a field success which will have the value true if the operation was a success and false otherwise. If success is false, the response object will contain a field error which will contain the JSON object. The error object will contain two fields errorType, a single word description of the error, and errorMsg, a string containing a description of the error.
The example code is written in Php and has two dependencies the Laminas library and Http_Request2.
The requirements to run examples given in the tutorial
- An installation of Simply with the web service;
- Php to run the example code;
- HTTP_Request2 which can be installed by doing a
pear install HTTP_Request2
; - Laminas, for which you will need to download the Laminas framework.
Logging in
The API does not use the password for logins. Instead, Simply provides a unique access key for each user. To get the user key, go to the My Preferences panel of a specific user. There, you will find the Access Key field.
Important: It is required to enter the IP in the Whitelist IP field under My Preferences on the user’s profile in Simply CRM that is being used to access the API.
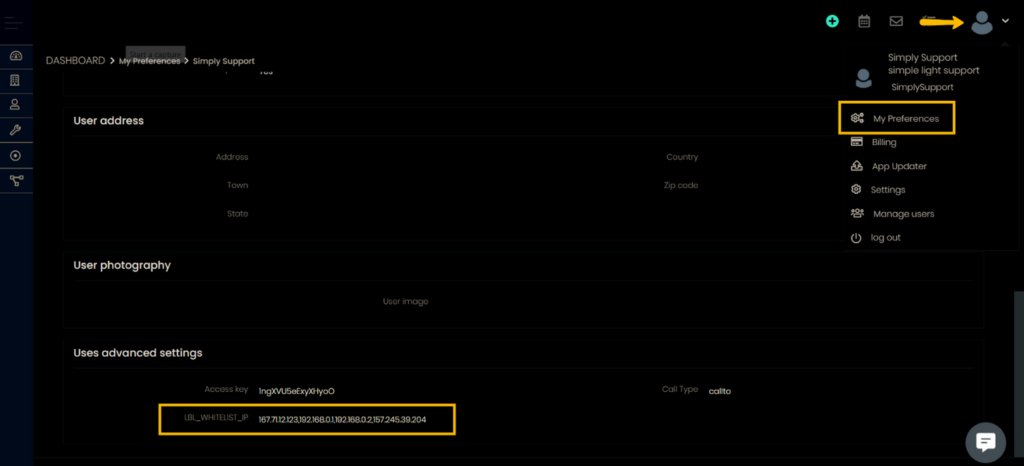
Each login starts a client session with the server, authenticates the user and returns a sessionId which will be used for all subsequent communication with the server.
Logging in is a two-step process. In the first step, the client obtains the challenge token from the server, which is used along with the user’s access key to log in.
GetChallenge
Get a challenge token from the server. This must be a GET request.
URL format
<code>Request Type: GET
http://Simply_url/webservice.php?operation=getchallenge
&username=[username]
</code>
GetChallenge example
To get the challenge token, you need to execute the following code.
<?php
require_once 'HTTP/Request2.php';
$endpointUrl = 'https://tr.simply-crm.dk/webservice.php';
//username of the user who is to logged in.
$userName="API";
try {
$request = new HTTP_Request2($endpointUrl . "?operation=getchallenge&username=" . $userName, HTTP_Request2::METHOD_GET);
$response = $request->send();
if (200 == $response->getStatus()) {
$jsonResponse = json_decode($response->getBody(),true);
$challengeToken = $jsonResponse['result']['token'];
echo $challengeToken;
} else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}catch (HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
?>
GetChallenge result
An object representing the response result of a getchallenge operation.
<code>GetChallengeResult{
token:String //Challenge token from the server.
serverTime:TimeStamp //The current server time.
expireTime:TimeStamp //The time when the token expires.
}
</code>
Now that you have the challenge token you can go ahead with the login. The access key field in the login operation is the md5 checksum of the concatenation of the challenge token and the user’s own access key. The login operation, as opposed to getchallenge, is a post request.
Login
Login to the server using the challenge token obtained in the getchallenge operation.
URL format
<code>Request Type:POST
http://Simply_url/webservice.php?operation=login
&username=[username]
&accessKey=[accessKey]
</code>
For accessKey, create a md5 string after concatenating user accesskey from the My Preference page and the challenge token obtained from the getchallenge result.
Login example
<code>//e.g.
//access key of the user admin, found on my preferences page.
$userAccessKey = 'dsf923rksdf3';
//create md5 string concatenating user accesskey from my preference page
//and the challenge token obtained from get challenge result.
$generatedKey = md5($challengeToken.$userAccessKey);
//login request must be POST request.
$httpc->post("$endpointUrl",
array('operation'=>'login', 'username'=>$userName,
'accessKey'=>$generatedKey), true);
$response = $httpc->currentResponse();
//decode the json encode response from the server.
$jsonResponse = Zend_JSON::decode($response['body']);
//operation was successful get the token from the reponse.
if($jsonResponse['success']==false)
//handle the failure case.
die('login failed:'.$jsonResponse['error']['errorMsg']);
//login successful extract sessionId and userId from LoginResult to it can used for further calls.
$sessionId = $jsonResponse['result']['sessionName'];
$userId = $jsonResponse['result']['userId'];
</code>
The session ID is used to identify the current session and will be a common parameter in all subsequent requests.
Login result
An object representing the response result of a login operation.
<code>LoginResult{
sessionId:String //Unique Identifier for the session
userId:String //The Simply id for the logged in user
version:String //The version of the webservices api
SimplyVersion:String //The version of the Simply crm.
}
</code>
Getting information
The API provides two operations to get information about the available Simply objects.
The first one is listtypes, which provides a list of available modules. This list only contains modules the logged in user has access to.
List types
List the names of all the Simply objects available through the API.
URL format
<code>Request Type: GET
http://Simply_url/webservice.php?operation=listtypes
&sessionName=[session id]
</code>
Returns a map containing the key ‘types’ with the value being a list of the Simply object names.
List types example
<code>//listtypes request must be GET request.
$httpc->get("$endpointUrl?sessionName=$sessionId&operation=listtypes");
$response = $httpc->currentResponse();
//decode the json encode response from the server.
$jsonResponse = Zend_JSON::decode($response['body']);
//operation was successful get the token from the reponse.
if($jsonResponse['success']==false)
//handle the failure case.
die('list types failed:'.$jsonResponse['error']['errorMsg']);
//Get the List of all the modules accessible.
$modules = $jsonResponse['result']['types'];
</code>
The second operation is describe and it provides detailed type information about a Simply object.
Describe
Get the type information about a given Simply object.
URL format
<code>Request Type: GET
http://Simply_url/webservice.php?operation=describe
&sessionName=[session id]
&elementType=[elementType]
</code>
Describe example
<code>//e.g.
//Simply Object name which need be described or whose information is requested.
$moduleName = 'Contacts';
//use sessionId created at the time of login.
$params = "sessionName=$sessionId&operation=describe&elementType=$moduleName";
//describe request must be GET request.
$httpc->get("$endpointUrl?$params");
$response = $httpc->currentResponse();
//decode the json encode response from the server.
$jsonResponse = Zend_JSON::decode($response['body']);
//operation was successful get the token from the reponse.
if($jsonResponse['success']==false)
//handle the failure case.
die('describe object failed:'.$jsonResponse['error']['errorMsg']);
//get describe result object. $description = $jsonResponse['result'];
</code>
The description consists of the following fields:
Name | Description |
Label | The label used for the name of the module. |
Name | The name of the module. |
Createable | A boolean value specifying whether the object can be created. |
Updateable | A boolean value specifying whether the object can be updated. |
Deleteable | A boolean value specifying whether the object can be deleted. |
Retrieveable | A boolean value specifying whether the object can be retrieved. |
Fields | An array containing the field names and their type information. |
Each element in the fields array describes a particular field in the object.
Name | Description |
Name | The name of the field, as used internally by Simply. |
Label | The label used for displaying the field name. |
Mandatory | A boolean that specifies whether the field is mandatory. Mandatory fields must be provided when creating a new object. |
Type | A map that describes the type information for the field. |
Default | The default value for the field. |
Nillable | A boolean that specifies whether the field can be set to null. |
Editable | A boolean that specifies whether the field can be modified. |
The type field is particularly important as it describes what type of the field is. This is a map that will contain at the least one element called name, which specifies the name of the type.
Name | Description |
String | A one line text field. |
Text | A multiline text field. |
Integer | A multiline text field. |
Double | A field for for floating point numbers. |
Boolean | A boolean field, which can have the values true or false. |
Time | A string in the format hh:mm, which is based on the user’s time format settings. |
Date | A string representing a date, the type map will contain another element called format which is the format in which the value of this field is expected, its based on the user’s settings date format. |
Datetime | A string representing the date and time, which is based on the user’s date format settings. |
Autogenerated | The fields for which the values are generated automatically by Simply. This is usually an object’s ID field. |
Reference | A field that shows a relation to another object. The type map will contain another element called refersTo, which is an array containing the modules to which the field can point. |
Picklist | A field that can a hold one of a list of values. The map will contain two elements, picklistValues, which is a list of possible values, and defaultValue, which is the default value for the picklist. |
Multipicklist | A picklist field where multiple values can be selected. |
Phone | A field used to store phone numbers. |
A field used to store email IDs. | |
URL | A field used to store URLs. |
Skype | A field used to store Skype IDs or phone numbers. |
Password | A field used to store passwords. |
Owner | A field that defines the owner of the field, which can be a group or an individual user. |
Describe result
<code>DescribeResult{
label:String //label of the module.
name:String //name of the module, as one provided in request.
createable:Boolean //true if the logged-in user is allowed create records of type and false otherwise.
updateable:Boolean //true if the logged-in user is allowed update records of type and false otherwise.
deleteable:Boolean //true if the logged-in user is allowed delete records of type and false otherwise.
retrieveable:Boolean //true if the logged-in user is allowed retrieveable records of type and false otherwise.
fields:Array //array of type Field.
}
</code>
Field
<code>Field{
<pre class="_fck_mw_lspace"> name:String //name of the field.
label:String //label of the field.
mandatory:Boolean //true if the needs to be provided with value while doing a create request and false otherwise.
type:FieldType //an instance of FieldType.
default:anyType //the default value for the field.
nillable:Boolean //true if null can provided a value for the field and false otherwise.
editable:Boolean //true if field can provided with a value while create or update operation.
}
</code>
FieldType
<code>FieldType{
<pre class="_fck_mw_lspace"> name:Type //field type
refersTo:Array //an array of Simply object names of types of record to which the field can refer to, its only defined for reference FieldType.
picklistValues:Array //an array of type PicklistValue type. its only defined for picklist Fieldtype.
defaultValue:String //an value of the default selected picklist value, its only defined for picklist Fieldtype.
format:String //date format in which date type field need to populated with, eg, mm-dd-yyyy. its only defined for Date Fieldtype.
}
</code>
The picklist field value needs to provided explicitly.
<code>PicklistValue{
name:String //name of the picklist option.
value:String //value of the picklist option.
}
</code>
A describeResult object example
//$description from the example above. For instance,print_r($description)
might display:
<code>Array
(
[label] => Contacts
[name] => Contacts
[createable] => 1
[updateable] => 1
[deleteable] => 1
[retrieveable] => 1
[fields] => Array
(
[0] => Array
(
[name] => account_id
[label] => Account Name
[mandatory] =>
[type] => Array
(
[name] => reference,
[refersTo] => Array
(
"Accounts"
)
)
[default] =>
[nillable] => 1
[editable] => 1
)
)
)
</code>
CRUD Operations
The API provides operations to create, retrieve, update and delete CRM entity objects.
Create
Create a new entry on the server.
URL format
<code>Request Type: POST
http://Simply_url/webservice.php?operation=create
&sessionName=[session id]
&element=[object]
&elementType=[object type]
</code>
Create example
Example 1
You can create a contact using the create operation. In this case, the mandatory fields are lastname and assigned_user_id.
<code>//e.g.
//fill in the details of the contacts.userId is obtained from loginResult.
$contactData = array('lastname'=>'Valiant', 'assigned_user_id'=>$userId);
//encode the object in JSON format to communicate with the server.
$objectJson = Zend_JSON::encode($contactData);
//name of the module for which the entry has to be created.
$moduleName = 'Contacts';
//sessionId is obtained from loginResult.
$params = array("sessionName"=>$sessionId, "operation"=>'create',
"element"=>$objectJson, "elementType"=>$moduleName);
//Create must be POST Request. $httpc->post("$endpointUrl", $params, true);
$response = $httpc->currentResponse();
//decode the json encode response from the server.
$jsonResponse = Zend_JSON::decode($response['body']);
//operation was successful get the token from the reponse.
if($jsonResponse['success']==false)
//handle the failure case.
die('create failed:'.$jsonResponse['error']['errorMsg']);
$savedObject = $jsonResponse['result'];
$id = $savedObject['id'];
</code>
$savedObject
is a map containing the fields of the new object including an ID field which can be used to refer to the object.
Example 2
Create a contact and associate it with an existing account.
<code>//e.g 2
//Create a Contact and associate with an existing Account.
$queryResult = doQuery("select accountname,id from accounts where accountname='Simply';");
$accountId = $queryResult[0]['id'];
//For more details on how do a query request please refer the query operation example.
//fill in the details of the contacts.userId is obtained from loginResult.
$contactData = array('lastname'=>'Valiant', 'assigned_user_id'=>$userId,'account_id'=>$accountId);
//encode the object in JSON format to communicate with the server.
$objectJson = Zend_JSON::encode($contactData);
//name of the module for which the entry has to be created.
$moduleName = 'Contacts';
//sessionId is obtained from loginResult.
$params = array("sessionName"=>$sessionId, "operation"=>'create',
"element"=>$objectJson, "elementType"=>$moduleName);
//Create must be POST Request.
$httpc->post("$endpointUrl", $params, true);
$response = $httpc->currentResponse();
//decode the json encode response from the server.
$jsonResponse = Zend_JSON::decode($response['body']);
//operation was successful get the token from the reponse.
if($jsonResponse['success']==false)
//handle the failure case.
die('create failed:'.$jsonResponse['error']['errorMsg']);
$savedObject = $jsonResponse['result'];
$id = $savedObject['id'];
</code>
Example 3
Create a contact and associate it with a new account.
<code>//e.g 3
<p>//Create a Contact and associate with a new Account.
</p><p>//fill in the details of the Accounts. userId is obtained from loginResult.
$accountData = array('accountname'=>'Simply', 'assigned_user_id'=>$userId);
//encode the object in JSON format to communicate with the server.
$objectJson = Zend_JSON::encode($accountData);
//name of the module for which the entry has to be created.
$moduleName = 'Accounts';
</p><p>//sessionId is obtained from loginResult.
$params = array("sessionName"=>$sessionId, "operation"=>'create',
</p>
<pre class="_fck_mw_lspace"> "element"=>$objectJson, "elementType"=>$moduleName);
//Create must be POST Request. $httpc->post(“$endpointUrl”, $params, true); $response = $httpc->currentResponse(); //decode the json encode response from the server. $jsonResponse = Zend_JSON::decode($response['body']);
//operation was successful get the token from the reponse.
if($jsonResponse['success']==false)
//handle the failure case.
die('create failed:'.$jsonResponse['error']['errorMsg']);
$account = $jsonResponse['result']; $accountId = $account['id'];
$contactData = array(‘lastname’=>’Valiant’, ‘assigned_user_id’=>$userId,’account_id’=>$accountId);
//encode the object in JSON format to communicate with the server. $objectJson = Zend_JSON::encode($contactData); //name of the module for which the entry has to be created. $moduleName = ‘Contacts’;
//sessionId is obtained from loginResult.
$params = array(“sessionName”=>$sessionId, “operation”=>’create’,
"element"=>$objectJson, "elementType"=>$moduleName);
//Create must be POST Request. $httpc->post(“$endpointUrl”, $params, true); $response = $httpc->currentResponse(); //decode the json encode response from the server. $jsonResponse = Zend_JSON::decode($response['body']);
//operation was successful get the token from the reponse.
if($jsonResponse['success']==false)
//handle the failure case.
die('create failed:'.$jsonResponse['error']['errorMsg']);
$savedObject = $jsonResponse['result']; $id = $savedObject['id'];
</code></pre>
CreateResult
A map representing the contents of a CRM entity based object. All reference fields are represented using ID type. A key called ID of type Id represents the object’s unique ID. This field is present for any object fetched from the database.
Retrieve
Retrieve an existing entry from the server.
URL format
<code>Request Type: GET
http://Simply_url/webservice.php?operation=retrieve
&session_name=[session id]
&id=[object id
</code>
Retrieve example
To retrieve an object using its ID, use the retrieve operation. You can retrieve the contact created in the create example.
<code>//sessionId is obtained from loginResult.
$params = "sessionName=$sessionId&operation=retrieve&id=$id";
//Retrieve must be GET Request.
$httpc->get("$endpointUrl?$params");
$response = $httpc->currentResponse();
//decode the json encode response from the server.
$jsonResponse = Zend_JSON::decode($response['body']);
//operation was successful get the token from the reponse.
if($jsonResponse['success']==false)
//handle the failure case.
die('retrieve failed:'.$jsonResponse['error']['errorMsg']);
$retrievedObject = $jsonResponse['result'];
</code>
RetrieveResult
A map representing the contents of a CRM entity based object. All reference fields are represented using Id type. A key called ID of type Id represents the object’s unique ID. This field is present for any object fetched from the database.
Update
To update a retrieved or newly created object, you can use the update operation.
URL format
<code>Request Type: POST
http://Simply_url/webservice.php?operation=update
&sessionName=[session id]
&element=[object]
</code>
Update example
You can add a first name to the contact.
<code>//retrievedObject from previous example(Example 1).
$retrievedObject['firstname'] = 'Prince';
//encode the object in JSON format to communicate with the server.
$objectJson = Zend_JSON::encode($retrievedObject);
//sessionId is obtained from the login operation result.
$params = array("sessionName"=>$sessionId, "operation"=>'update',
"element"=>$objectJson);
//update must be a POST request.
$httpc->post("$endpointUrl", $params, true);
$response = $httpc->currentResponse();
//decode the json encode response from the server.
$jsonResponse = Zend_JSON::decode($response['body']);
//operation was successful get the token from the reponse. if($jsonResponse['success']==false)
//handle the failure case.
die('update failed:'.$jsonResponse['error']['errorMsg']);
//update result object.
$updatedObject = $jsonResponse['result'];
</code>
UpdateResult
A map representing the contents of a crmentity based object. All reference fields are represented using Id type. A key called ID of type Id represents the object’s unique ID. This field is present for any object fetched from the database.
Delete
To delete an object, use the delete operation.
URL format
<code>Request Type: POST
http://Simply_url/webservice.php?operation=delete
&sessionName=[session id]
&id=[object id]
</code>
Delete example
<code>
//delete a record created in above examples, sessionId a obtain from the login result.
$params = array("sessionName"=>$sessionId, "operation"=>'delete', "id"=>$id);
//delete operation request must be POST request.
$httpc->post("$endpointUrl", $params, true);
$response = $httpc->currentResponse();
//decode the json encode response from the server.
$jsonResponse = Zend_JSON::decode($response['body']);
//operation was successful get the token from the reponse. if($jsonResponse['success']==false)
//handle the failure case.
die('delete failed:'.$jsonResponse['error']['errorMsg']);
</code>
The delete operation does not have a result. The success flag is enough to find out if the operation was successful.
Queries
Simply provides a simple query language for fetching data. This language is quite similar to select queries in SQL. There are limitations: the queries work on a single module, embeded queries are not supported, and it does not support joins. But this is still a powerful way of getting data from Simply.
Query always limits its output to 100 records. The client application can use the limit operator to get different records.
URL format
<code>Request Type: GET
http://Simply_url/webservice.php?operation=query
&sessionName=[session id]
&query=[query string]
</code>
Query example
Example 1
<code>//query to select data from the server.
$query = "select * from Contacts where lastname='Valiant';";
//urlencode to as its sent over http.
$queryParam = urlencode($query);
//sessionId is obtained from login result.
$params = "sessionName=$sessionId&operation=query&query=$queryParam";
//query must be GET Request.
$httpc->get("$endpointUrl?$params");
$response = $httpc->currentResponse();
//decode the json encode response from the server.
$jsonResponse = Zend_JSON::decode($response['body']);
//operation was successful get the token from the reponse. if($jsonResponse['success']==false)
//handle the failure case.
die('query failed:'.$jsonResponse['errorMsg']);
//Array of SimplyObjects
$retrievedObjects = $jsonResponse['result'];
</code>
Example 2
Specify the columns to be fetched for each record.
<code>//query to select data from the server.
$query = "select lastname,firstname,account_id,assigned_user_id from Contacts where lastname='Valiant';";
//urlencode to as its sent over http.
$queryParam = urlencode($query);
//sessionId is obtained from login result.
$params = "sessionName=$sessionId&operation=query&query=$queryParam";
//query must be GET Request.
$httpc->get("$endpointUrl?$params");
$response = $httpc->currentResponse();
//decode the json encode response from the server.
$jsonResponse = Zend_JSON::decode($response['body']);
//operation was successful get the token from the reponse.
if($jsonResponse['success']==false)
//handle the failure case.
die('query failed:'.$jsonResponse['errorMsg']);
//Array of SimplyObjects
$retrievedObjects = $jsonResponse['result'];
</code>
This will return an array containing arrays which represent the fields of each object that matched the query.
QueryResult
QueryResult is an array of SimplyObjects.
SimplyObject
A map representing the contents of a crmentity based object. All reference fields are represented using Id type. A key called ID of type Id represents the object’s unique ID. This field is present for any object fetched from the database.
Query format
<code>select * | <column_list> | <count(*)>
from <object> [where <conditionals>]
[order by <column_list>] [limit [<m>, ]<n>];
</code>
The column list in the order by clause can have at most two column names.
Name | Description |
column_list | A comma separated list of field names. |
object | The type name of the object. |
conditionals | The conditional operations or in clauses or like clauses separated by ‘and’ or ‘or’ operations these are processed from left to right. There is no grouping, that is bracket operators. |
conditional operators | <, >, <=, >=, =, != |
in clauses | <column name> in (<value list>). |
like clauses | <column name> in (<value pattern>). |
value list | A comma separated list of values. |
m, n | The integer values to specify the offset and limit respectively. |
Sync
Sync will return a SyncResult object containing the details of the changes after modifiedTime.
URL format
<code>Request Type: GET
http://Simply_url/webservice.php?operation=sync
&sessionName=[session id]
&modifiedTime=[timestamp]
&elementType=[elementType]
</code>
elementType – This is an optional parameter. If specified only the changes for that module after the given time are returned. Otherwise, the changes to all user accessible modules after the given time are returned.
TimeStamp – A long representation of the number of seconds since Unix epoch.
Example 1
Create an account and capture it in the sync API.
<code>//time after which all the changes on the server are needed.
$stime = time();
//Create some data now so it is captured by the sync api response.
//Create Account.
//fill in the details of the Accounts.userId is obtained from loginResult.
$accountData = array('accountname'=>'Simply', 'assigned_user_id'=>$userId);
//encode the object in JSON format to communicate with the server. $objectJson = Zend_JSON::encode($accountData);
//name of the module for which the entry has to be created.
$moduleName = 'Accounts';
//sessionId is obtained from loginResult.
$params = array("sessionName"=>$sessionId, "operation"=>'create',
"element"=>$objectJson, "elementType"=>$moduleName);
//Create must be POST Request.
$httpc->post("$endpointUrl", $params, true);
$response = $httpc->currentResponse();
//decode the json encode response from the server.
$jsonResponse = Zend_JSON::decode($response['body']);
//operation was successful get the token from the reponse.
if($jsonResponse['success']==false)
//handle the failure case.
die('create failed:'.$jsonResponse['error']['errorMsg']);
$createResult = $jsonResponse['result'];
$params = "operation=sync&modifiedTime=$stime&sessionName=$sessionId";
//sync must be GET Request.
$httpc->get("$endpointUrl?$params");
$response = $httpc->currentResponse();
//decode the json encode response from the server.
$jsonResponse = Zend_JSON::decode($response['body']);
//operation was successful get the token from the reponse.
if($jsonResponse['success']==false)
//handle the failure case.
die('query failed:'.$jsonResponse['errorMsg']);
//Array of SimplyObjects
$retrievedObjects = $jsonResponse['result'];
</code>
The response contains the account that was created.
Example 2
Create an account and a contact, use the sync API only for the Accounts module, only changes to Accounts module are returned.
<code>//time after which all the changes on the server are needed.
$stime = time();
//Create some data now so it is captured by the sync api response.
//fill in the details of the Accounts.userId is obtained from loginResult.
$accountData = array('accountname'=>'Simply', 'assigned_user_id'=>$userId);
//encode the object in JSON format to communicate with the server.
$objectJson = Zend_JSON::encode($accountData);
//name of the module for which the entry has to be created.
$moduleName = 'Accounts';
//sessionId is obtained from loginResult.
$params = array("sessionName"=>$sessionId, "operation"=>'create',
"element"=>$objectJson, "elementType"=>$moduleName);
//Create must be POST Request.
$httpc->post("$endpointUrl", $params, true);
$response = $httpc->currentResponse();
//decode the json encode response from the server.
$jsonResponse = Zend_JSON::decode($response['body']);
//operation was successful get the token from the reponse.
if($jsonResponse['success']==false)
//handle the failure case.
die('create failed:'.$jsonResponse['error']['errorMsg']);
$createResult = $jsonResponse['result'];
//Create a Contact.
//fill in the details of the contacts.userId is obtained from loginResult.
$contactData = array('lastname'=>'Valiant', 'assigned_user_id'=>$userId);
//encode the object in JSON format to communicate with the server.
$objectJson = Zend_JSON::encode($contactData);
//name of the module for which the entry has to be created.
$moduleName = 'Contacts';
//sessionId is obtained from loginResult.
$params = array("sessionName"=>$sessionId, "operation"=>'create',
"element"=>$objectJson, "elementType"=>$moduleName);
//Create must be POST Request.
httpc->post("$endpointUrl", $params, true);
$response = $httpc->currentResponse();
//decode the json encode response from the server.
$jsonResponse = Zend_JSON::decode($response['body']);
//operation was successful get the token from the reponse.
if($jsonResponse['success']==false)
//handle the failure case.
die('create failed:'.$jsonResponse['error']['errorMsg']);
$savedObject = $jsonResponse['result'];
$id = $savedObject['id'];
$params = "operation=sync&modifiedTime=$stime&sessionName=$sessionId";
//sync must be GET Request.
$httpc->get("$endpointUrl?$params");
$response = $httpc->currentResponse();
//decode the json encode response from the server.
$jsonResponse = Zend_JSON::decode($response['body']);
//operation was successful get the token from the reponse.
if($jsonResponse['success']==false)
//handle the failure case.
die('query failed:'.$jsonResponse['errorMsg']);
//Array of SimplyObjects
$retrievedObjects = $jsonResponse['result'];
</code>
SyncResult
An object representing the response of a sync operation.
<code>SyncResult{
updated:[Object] //List of Objects created or modified.
deleted:[Id] //List of *Id* of objects deleted.
lastModifiedTime:Timstamp //time of the latest change. which can used in the next call to the Sync api to get all the latest changes that the client hasn't obtained.
}
</code>
Logging Out
Logout
Log out from the web service session, which leaves the webservice session invalid for furthur use.
URL format
<code>Request Type: GET
http://Simply_url/webservice.php?operation=logout
&sessionName=[session id]
</code>
Example
<code>//SessionId is the session which is to be terminated.
$params = "operation=logout&sessionName=$sessionId";
//logout must be GET Request.
$httpc->get("$endpointUrl?$params");
$response = $httpc->currentResponse();
//decode the json encode response from the server.
$jsonResponse = Zend_JSON::decode($response['body']);
//operation was successful get the token from the reponse.
if($jsonResponse['success']==false)
//handle the failure case.
die('query failed:'.$jsonResponse['errorMsg']);
//logout successful session terminated.
</code>
Extending the Simply web client using web service
Extend session
Extends the current Simply web client session to the web service and returns a webservice session ID. When working the from within the Simply web client, the user can re-use the current authenticated session instead of logging in to Simply again to access the web service.
Note: If the user extends a session, then the session will be tied together, so logging out from one (the web service or the web client) will log the user out of the other as well. For the extend session operation to work, cookies must be enabled on the client browser.
URL format
<code>Request Type: POST
http://Simply_url/webservice.php?operation=extendsession
</code>
Example
<code>
</code>
This example is written is Javascript, as the Extend Session is only valid from the Simply web client.
Requirements to run the example
- JQUERY library – to do the ajax http request.
- JSON library – json2.js file available with Simply at json.org.
Example 1
<code>
//operation parameters.
var params = "operation=extendsession";
//end point of the services.
var endPointUrl = "http://Simply_url/webservice.php";
//extendSession is post request, invoke jquery http post request.
$.post(endPointUrl, params, function(result){
//decode the json encode response from the server.
var jsonResponse = json.parse(result);
//operation was successful get the token from the reponse.
if(jsonResponse['success']==false)
//handle the failure case.
die('login failed:'+jsonResponse['error']['errorMsg']);
//login successful extract sessionId and userId from LoginResult so it can used for further calls.
var sessionId = jsonResponse['result']['sessionName'];
var userId = jsonResponse['result']['userId'];
});
</code>